- Published at
Introducing SwiftTesting
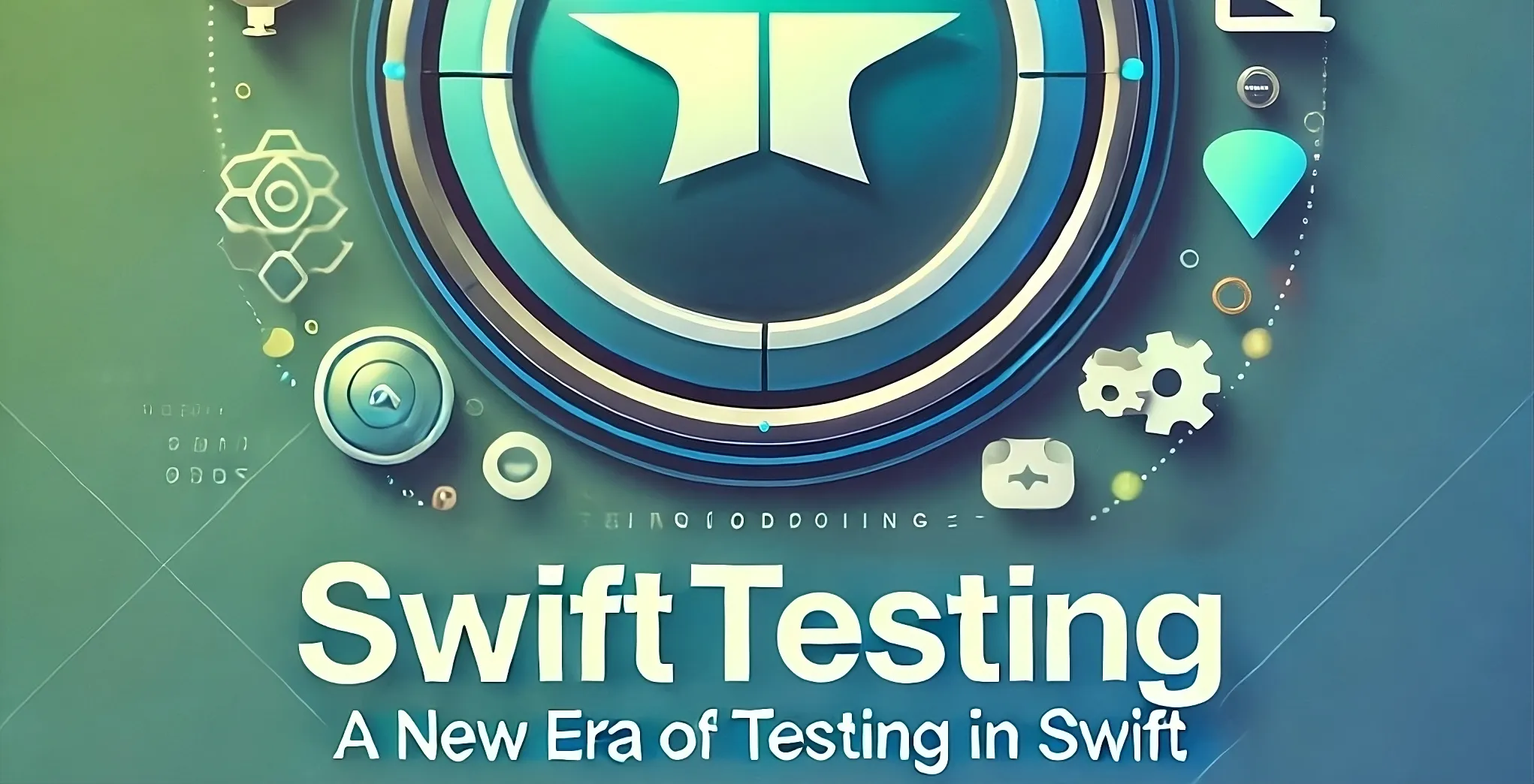
Discover the new SwiftTesting framework and how it revolutionizes testing in Swift with simplified syntax and enhanced functionality.
- Authors
-
-
- Name
- Andy Kolean
-
Table of Contents
- 1. Introduction
- 2. What is SwiftTesting?
- Key Motivations Behind SwiftTesting
- Differences Between SwiftTesting and XCTest
- 3. Getting Started with SwiftTesting
- Setting Up SwiftTesting
- Basic Syntax and Structure
- Example: Full Test Suite
- Handling Setup and Teardown
- 4. Key Features and Advantages
- Parallel Execution
- Randomized Test Execution
- Simplified Assertions with #expect
- Organizing Tests with @Suite
- 5. Detailed Examples
- Basic Test Case
- Simplified Assertions
- Using Suites
- Detailed Suite Example
- Combining Tests and Suites
- 6. Advanced Features
- Traits (Description, Tag, Bug, Toggle, Time Limit)
- Parametrized Tests
- Testing Async Code
- Validating Errors
- 7. Error Handling in SwiftTesting
- Introduction to Issue.record
- Validating Failures
- Handling Optional Values
- Recording Issues Without Failing the Test
- Combining Issue.record with #expect
- Handling Multiple Issues
- 8. Advanced Test Management
- Test Plans
- Test Observers
- Custom Test Execution
- Detailed Test Reporting
- 9. Compatibility
- Supported Platforms
- Version Compatibility
- Example of Version-Specific Features
- 10. Comparing XCTest and SwiftTesting
- Side-by-Side Code Comparison
- Performance Improvements
- Simplified Syntax and Organization
- Migrating from XCTest to SwiftTesting
- 11. Conclusion
- Key Takeaways
- Next Steps
- References and Further Reading
1. Introduction
In this blog post, we introduce SwiftTesting, Apple’s new testing framework announced at WWDC24. SwiftTesting promises to revolutionize the way we write and execute tests for Swift applications, offering a more robust and streamlined approach compared to the traditional XCTest framework. We’ll explore the key features, advantages, and provide detailed examples to help you get started with SwiftTesting.
Apple has a long history of innovation in software development, and SwiftTesting is the latest addition to their toolkit aimed at improving the developer experience. As software becomes more complex, the need for efficient and reliable testing frameworks grows. SwiftTesting addresses these needs with modern features and a more intuitive approach to writing tests.
2. What is SwiftTesting?
SwiftTesting is a modern testing framework designed by Apple to simplify and enhance the testing experience for Swift developers. Unlike XCTest, SwiftTesting is built with advanced features like parallel and randomized test execution by default, which ensures more efficient and reliable testing processes.
Key Motivations Behind SwiftTesting
- Efficiency: SwiftTesting aims to reduce the complexity of writing and maintaining tests. With its simplified syntax and powerful features, developers can write tests faster and with less boilerplate code.
- Performance: With support for parallel and randomized test execution, tests run faster and detect flaky tests more effectively. This leads to a more robust test suite and quicker feedback during development.
- Simplicity: The new syntax and structure make it easier to write and organize tests. SwiftTesting uses macros like
@Test
and#expect
to streamline test creation, making it more intuitive for developers.
Differences Between SwiftTesting and XCTest
- Test Declaration: SwiftTesting uses the
@Test
macro for defining tests, while XCTest relies on test methods withinXCTestCase
. This reduces the boilerplate and makes test functions more concise. - Assertions: SwiftTesting simplifies assertions with the
#expect
macro, replacing the extensiveXCTAssert
functions. This unifies all assertions under a single function, making the code cleaner and easier to read. - Test Organization: SwiftTesting introduces the
@Suite
macro for organizing tests, providing more flexibility compared to XCTest’s class-based structure. This allows for better modularity and separation of test concerns.
3. Getting Started with SwiftTesting
Getting started with SwiftTesting is straightforward. This section will guide you through setting up SwiftTesting in your project and explain the basic syntax and structure you’ll need to get up and running.
Setting Up SwiftTesting
To integrate SwiftTesting into your project, you need to import the Testing library and begin writing your tests using the new syntax provided by SwiftTesting.
-
Import the Testing Module
The first step is to import the Testing module into your Swift file. This replaces the traditional XCTest import.
import Testing
-
Writing a Basic Test Function
In SwiftTesting, you declare test functions using the
@Test
macro. This replaces the need to inherit fromXCTestCase
and use specific naming conventions for test methods.@Test func myFirstTest() { let value = 2 + 2 #expect(value == 4) }
-
Organizing Tests with Suites
SwiftTesting allows you to group related tests using the
@Suite
macro. This provides a clean and modular way to organize your test functions.@Suite struct MathTests { @Test func testAddition() { let result = 1 + 1 #expect(result == 2) } }
Basic Syntax and Structure
The basic syntax and structure of SwiftTesting are designed to be intuitive and concise. Here’s a quick overview of the main components you’ll use when writing tests:
- @Test: This macro is used to declare a test function. It can be applied to any function that you want to be recognized as a test. Unlike XCTest, there’s no need for a specific naming convention.
- #expect: This macro is used for assertions. It replaces the various
XCTAssert
functions in XCTest, providing a unified way to perform assertions. It simplifies the assertion process and makes the code more readable. - @Suite: This macro is used to group related tests into a suite. It can be applied to structs, classes, enums, and actors. This allows for better modularity and separation of test concerns.
Example: Full Test Suite
Here’s a more comprehensive example that demonstrates a full test suite using SwiftTesting:
import Testing
@Suite struct CalculatorTests {
var calculator: Calculator
init() {
calculator = Calculator()
}
@Test func testAddition() {
let result = calculator.add(2, 3)
#expect(result == 5)
}
@Test func testSubtraction() {
let result = calculator.subtract(5, 3)
#expect(result == 2)
}
@Test func testMultiplication() {
let result = calculator.multiply(2, 3)
#expect(result == 6)
}
@Test func testDivision() throws {
let result = try calculator.divide(6, 3)
#expect(result == 2)
}
}
In this example, CalculatorTests
is a suite containing four test functions: testAddition
, testSubtraction
, testMultiplication
, and testDivision
. Each test function performs an operation using the Calculator
instance and verifies the result using the #expect
macro. The testDivision
function also demonstrates handling errors with a throws
clause.
Handling Setup and Teardown
In SwiftTesting, setup and teardown functions are handled using initializers (init
) and deinitializers (deinit
) instead of setUp
and tearDown
methods in XCTest. This ensures that each test starts with a clean state.
@Suite struct CalculatorTests {
var calculator: Calculator
init() {
calculator = Calculator()
// Additional setup code
}
deinit {
// Code to run after each test
}
@Test func testAddition() {
let result = calculator.add(2, 3)
#expect(result == 5)
}
}
Using init
and deinit
for setup and teardown helps maintain test isolation and ensures that tests do not share state unintentionally.
4. Key Features and Advantages
SwiftTesting introduces several advanced features designed to enhance the testing experience for Swift developers. These features aim to improve test efficiency, reliability, and simplicity. Here’s a detailed look at the key features and advantages of SwiftTesting.
Parallel Execution
One of the standout features of SwiftTesting is its support for parallel execution. By default, tests in SwiftTesting are executed in parallel, both for synchronous and asynchronous tests. This can significantly reduce the overall time required to run your test suite, especially as the number of tests grows.
Benefits:
- Speed: Parallel execution allows multiple tests to run simultaneously, making the testing process faster.
- Efficiency: Utilizes multi-core processors more effectively, ensuring that CPU resources are not wasted.
- Scalability: Handles large test suites more efficiently, reducing the bottleneck of sequential test execution.
Example:
@Suite struct ParallelTests {
@Test func testOne() {
// Some test code
#expect(true)
}
@Test func testTwo() {
// Some other test code
#expect(true)
}
}
In this example, testOne
and testTwo
can be executed in parallel, reducing the overall test execution time.
Randomized Test Execution
SwiftTesting also supports randomized test execution by default. This feature helps in identifying flaky tests that might pass or fail depending on the order of execution or the environment state.
Benefits:
- Reliability: Ensures that tests are not dependent on the order of execution.
- Flaky Test Detection: Helps identify tests that fail intermittently due to dependencies on other tests.
- Improved Test Isolation: Encourages writing tests that are more isolated and independent.
Example:
@Suite struct RandomTests {
@Test func testA() {
// Test code A
#expect(true)
}
@Test func testB() {
// Test code B
#expect(true)
}
}
With randomized execution, testA
and testB
will run in different orders in different test runs, helping detect any hidden dependencies between them.
Simplified Assertions with #expect
The #expect
macro in SwiftTesting consolidates various assertion types into a single, easy-to-use function. This simplifies the syntax and makes the test code more readable.
Benefits:
- Unified Assertions: Replaces multiple
XCTAssert
functions with a single#expect
function. - Readability: Makes the code cleaner and easier to understand.
- Consistency: Provides a consistent way to write assertions across all tests.
Example:
@Test func testAssertions() {
let isTrue = true
#expect(isTrue)
let number = 5
#expect(number > 3)
}
In this example, #expect
is used to perform assertions, replacing traditional XCTAssert
functions.
Organizing Tests with @Suite
The @Suite
macro allows developers to group related tests into a suite. This provides better organization and modularity, making it easier to manage large test suites.
Benefits:
- Modularity: Groups related tests together, making the test structure more modular.
- Readability: Improves the readability and maintainability of the test suite.
- Flexibility: Supports different Swift types (structs, classes, enums, actors) for organizing tests.
Example:
@Suite struct CalculatorTests {
@Test func testAddition() {
let result = 2 + 3
#expect(result == 5)
}
@Test func testSubtraction() {
let result = 5 - 3
#expect(result == 2)
}
}
In this example, CalculatorTests
is a suite that groups two related tests: testAddition
and testSubtraction
.
5. Detailed Examples
To illustrate the power and simplicity of SwiftTesting, we’ll walk through several examples that demonstrate its key features and syntax. These examples include basic test cases, simplified assertions, and organizing tests with suites.
Basic Test Case
Let’s start with a basic test case that verifies a simple mathematical operation. In this example, we’ll test whether the sum of two numbers equals four.
import Testing
@Test func testInitialization() {
let value = 2 + 2
#expect(value == 4)
}
In this example:
- We use the
@Test
macro to define a test function namedtestInitialization
. - The
#expect
macro is used to assert that the value of2 + 2
equals4
.
This simple example showcases how straightforward it is to write tests with SwiftTesting compared to XCTest.
Simplified Assertions
SwiftTesting simplifies the assertion process by consolidating various XCTAssert
functions into a single #expect
macro. This makes the test code cleaner and easier to read.
@Test func testBoolean() {
let isTrue = true
#expect(isTrue)
}
Here, the #expect
macro checks whether the variable isTrue
is indeed true
.
Another example with multiple assertions:
@Test func testMultipleAssertions() {
let number = 5
#expect(number > 3)
#expect(number < 10)
}
In this example:
- The
#expect
macro is used twice to check different conditions, making the code concise and readable.
Using Suites
The @Suite
macro allows us to group related tests into a single suite. This helps in organizing tests better, especially when dealing with larger projects.
@Suite struct ArithmeticTests {
@Test func testAddition() {
let result = 1 + 1
#expect(result == 2)
}
@Test func testSubtraction() {
let result = 3 - 1
#expect(result == 2)
}
}
In this example:
- The
ArithmeticTests
suite contains two test functions:testAddition
andtestSubtraction
. - Both tests verify basic arithmetic operations using the
#expect
macro.
Detailed Suite Example
Let’s look at a more comprehensive suite example, demonstrating setup and teardown using initializers and deinitializers, and including multiple types of tests.
import Testing
@Suite struct CalculatorTests {
var calculator: Calculator
init() {
calculator = Calculator()
// Additional setup code
}
deinit {
// Code to run after each test
}
@Test func testAddition() {
let result = calculator.add(2, 3)
#expect(result == 5)
}
@Test func testSubtraction() {
let result = calculator.subtract(5, 3)
#expect(result == 2)
}
@Test func testMultiplication() {
let result = calculator.multiply(2, 3)
#expect(result == 6)
}
@Test func testDivision() throws {
let result = try calculator.divide(6, 3)
#expect(result == 2)
}
}
In this example:
CalculatorTests
is a suite that groups multiple test functions.- The
init
method sets up the test environment by initializing aCalculator
instance. - The
deinit
method can be used for cleanup after each test. - Multiple test functions (
testAddition
,testSubtraction
,testMultiplication
,testDivision
) test different calculator operations.
Combining Tests and Suites
To further illustrate the flexibility of SwiftTesting, let’s combine tests and suites in a more complex structure. This demonstrates how you can organize tests hierarchically.
@Suite struct MathOperationsTests {
@Suite struct AdditionTests {
@Test func testPositiveNumbers() {
let result = 2 + 3
#expect(result == 5)
}
@Test func testNegativeNumbers() {
let result = -2 + -3
#expect(result == -5)
}
}
@Suite struct SubtractionTests {
@Test func testPositiveNumbers() {
let result = 5 - 2
#expect(result == 3)
}
@Test func testNegativeNumbers() {
let result = -5 - -2
#expect(result == -3)
}
}
}
In this example:
MathOperationsTests
is the top-level suite containing nested suitesAdditionTests
andSubtractionTests
.- Each nested suite contains tests related to specific operations (addition or subtraction).
6. Advanced Features
SwiftTesting introduces several advanced features to make testing more powerful and flexible. Here we’ll look at traits, parametrized tests, testing async code, and validating errors in more detail.
Traits (Description, Tag, Bug, Toggle, Time Limit)
Traits add metadata to your tests, providing additional context and control. You can use traits to describe tests, tag them for filtering, link to bug reports, toggle test execution, and set time limits.
Description Trait: Provides a human-readable description for the test, which can be helpful in understanding the purpose of the test.
@Test("Simple Addition Test")
func testAddition() {
let result = 1 + 1
#expect(result == 2)
}
Tag Trait: Tags can be used to categorize tests. Tags help in filtering and organizing tests, especially in large test suites.
@Test(.tags(.math, .arithmetic))
func testAddition() {
let result = 1 + 1
#expect(result == 2)
}
Bug Trait: Link a test to a bug report to keep track of known issues and their related tests. This is useful for documentation and tracking the progress of bug fixes.
@Test(.bug("https://bugtracker.com/issue/123", "Fix issue with addition"))
func testAddition() {
let result = 1 + 1
#expect(result == 2)
}
Toggle Trait: Enable or disable tests based on runtime conditions, such as feature flags or configuration settings.
@Test(.enabled(if: FeatureFlag.isFeatureEnabled))
func testNewFeature() {
#expect(Feature.isActive)
}
Time Limit Trait: Set a maximum execution time for a test. This helps in identifying performance regressions and ensuring that tests complete within acceptable time frames.
@Test(.timeLimit(.seconds(1)))
func testPerformance() {
let start = Date()
performIntensiveTask()
let duration = Date().timeIntervalSince(start)
#expect(duration < 1)
}
Parametrized Tests
SwiftTesting allows you to run the same test with different parameters using parametrized tests. This reduces code duplication and makes tests more flexible.
Basic Parametrized Test:
@Test(
"Addition Test",
arguments: [1, 2, 3, 4]
)
func testAddition(_ number: Int) {
let result = number + number
#expect(result == number * 2)
}
In this example:
- The
testAddition
function runs four times, each time with a different value from thearguments
array. - The test checks if doubling the input number produces the correct result.
Multiple Parameters Example:
You can also pass multiple parameters to a test function, making it even more versatile.
@Test(
"Arithmetic Operations Test",
arguments: [
(2, 3, 5),
(4, 1, 5),
(3, 2, 5)
]
)
func testAddition(_ a: Int, _ b: Int, _ expected: Int) {
let result = a + b
#expect(result == expected)
}
In this example:
- The
testAddition
function runs three times with different sets of arguments. - It checks if the sum of the first two arguments equals the third argument.
Testing Async Code
SwiftTesting supports async tests, making it easy to test asynchronous code. You simply mark the test function with async
and use await
as needed.
Async Testing Example:
@Test func fetchData() async {
let data = await fetchDataFromServer()
#expect(data != nil)
}
In this example:
- The
fetchData
test function is marked withasync
. - The test waits for the
fetchDataFromServer
function to complete and then asserts that the result is not nil.
Complex Async Example:
Testing more complex asynchronous interactions is also straightforward.
@Test func fetchDataAndProcess() async {
let data = await fetchDataFromServer()
#expect(data != nil)
let processedData = processData(data!)
#expect(processedData.isValid)
}
In this example:
- The test fetches data asynchronously, then processes the data, and checks the validity of the processed data.
Validating Errors
SwiftTesting simplifies error handling in tests. You can use the #expect
macro to check for thrown errors.
Basic Error Validation:
@Test("Testing error throwing")
func testError() throws {
#expect(throws: MyError.exampleError.self) {
throw MyError.exampleError
}
}
In this example:
- The
testError
function throws an error, and the#expect
macro verifies that the correct error type is thrown.
Validating No Errors:
You can also ensure that no errors are thrown during a test.
@Test("Ensuring no errors thrown")
func testNoError() throws {
#expect(throws: Never.self) {
try someFunctionThatShouldNotThrow()
}
}
In this example:
- The
testNoError
function checks thatsomeFunctionThatShouldNotThrow
does not throw any errors.
Custom Error Handling:
SwiftTesting allows for more detailed error handling and checking specific properties of thrown errors.
enum NetworkError: Error {
case timeout
case serverError(code: Int)
}
@Test("Testing network error")
func testNetworkError() throws {
#expect(throws: NetworkError.serverError(code: 500)) {
throw NetworkError.serverError(code: 500)
}
}
In this example:
- The
testNetworkError
function checks for a specific error type and error value, demonstrating how to handle more complex error scenarios.
7. Error Handling in SwiftTesting
SwiftTesting introduces a new way to handle errors during tests, making it more streamlined and integrated compared to traditional XCTest. Here we explore how to record and manage issues within your tests using Issue.record
.
Introduction to Issue.record
The Issue.record
function allows you to document and handle issues directly within your test code. This function serves a similar purpose to XCTFail
in XCTest but is more integrated into the SwiftTesting framework.
Basic Usage:
@Test func testWithIssue() {
Issue.record("This is a recorded issue")
#expect(true == false)
}
In this example:
- The
Issue.record
function is used to log an issue with a descriptive message. - The
#expect
macro is then used to perform an assertion that will fail, demonstrating how the issue is recorded.
Validating Failures
When a test needs to fail due to a specific condition or check, Issue.record
provides a clear and descriptive way to document the failure.
Example of a Conditional Failure:
@Test func testConditionalFailure() {
let condition = false
if !condition {
Issue.record("Condition is false, expected true")
}
#expect(condition)
}
In this example:
- The condition is checked, and if it is false, an issue is recorded with a descriptive message.
- The
#expect
macro then verifies the condition, which will fail and confirm the recorded issue.
Handling Optional Values
SwiftTesting provides a convenient way to handle optional values and ensure they are not nil, using the #require
macro. This replaces the need for XCTUnwrap
.
Example of Unwrapping Optionals:
@Test func testUnwrappingOptional() {
let optionalValue: Int? = 5
let value = try! #require(optionalValue)
#expect(value == 5)
}
In this example:
- The
#require
macro is used to unwrap the optional value. If the value is nil, an error is thrown. - The unwrapped value is then verified using the
#expect
macro.
Recording Issues Without Failing the Test
Sometimes, you may want to record an issue without immediately failing the test. This is useful for logging known issues or potential problems that need to be addressed later.
Example of Logging an Issue:
@Test func testLoggingIssue() {
let result = performOperation()
if result == .unexpected {
Issue.record("Unexpected result encountered")
}
#expect(result != .unexpected)
}
In this example:
- An issue is recorded if the result is unexpected, but the test continues to check the result using the
#expect
macro.
Combining Issue.record
with #expect
You can combine Issue.record
with #expect
to provide more context and detail about the failure conditions in your tests.
Example of Combined Usage:
@Test func testDetailedFailure() {
let result = performOperation()
if result != .expected {
Issue.record("Operation did not return expected result")
}
#expect(result == .expected)
}
In this example:
- The issue is recorded with a descriptive message if the result is not as expected.
- The
#expect
macro then performs the actual assertion, providing a clear and detailed failure message if the test fails.
Handling Multiple Issues
SwiftTesting allows for handling multiple issues within a single test, giving you the flexibility to document and manage multiple failure points.
Example of Multiple Issues:
@Test func testMultipleIssues() {
let result1
= performFirstOperation()
if result1 != .expected {
Issue.record("First operation failed")
}
#expect(result1 == .expected)
let result2 = performSecondOperation()
if result2 != .expected {
Issue.record("Second operation failed")
}
#expect(result2 == .expected)
}
In this example:
- Multiple operations are performed, and issues are recorded for each one if they do not return the expected results.
- The
#expect
macro is used to perform assertions for each result, providing detailed failure messages if any of the checks fail.
8. Advanced Test Management
SwiftTesting offers advanced test management features that help you organize, control, and monitor your tests more effectively. This section explores test plans, test observers, and other advanced management techniques.
Test Plans
Test plans allow you to configure and control the execution of your tests in a detailed manner. They provide a structured way to define which tests should run under various conditions, set priorities, and handle different configurations.
Key Features of Test Plans:
- Configuration Management: Define different configurations for your tests, such as different environments or setups.
- Selective Test Execution: Specify which tests to run based on conditions or tags.
- Prioritization: Set the priority of tests to ensure critical tests run first.
Example Test Plan Configuration:
// This is a conceptual example as the exact implementation of test plans
// would depend on the specifics provided by SwiftTesting or other tools.
let testPlan = TestPlan {
$0.includeTags(["Critical", "LoginTests"])
$0.excludeTags(["Flaky"])
$0.setPriority("High", for: ["Critical"])
}
In this example:
- The test plan includes tests tagged with “Critical” and “LoginTests”.
- Tests tagged as “Flaky” are excluded.
- Tests with the “Critical” tag are given high priority.
Test Observers
Test observers allow you to hook into the test execution lifecycle, providing custom behavior at various stages of the testing process. This can be useful for logging, performance monitoring, or integrating with other tools.
Implementing a Test Observer:
class MyTestObserver: TestObserver {
func testSuiteWillStart(_ suite: TestSuite) {
print("Suite started: \(suite.name)")
}
func testSuiteDidFinish(_ suite: TestSuite) {
print("Suite finished: \(suite.name)")
}
func testCaseWillStart(_ testCase: TestCase) {
print("Test case started: \(testCase.name)")
}
func testCaseDidFinish(_ testCase: TestCase) {
print("Test case finished: \(testCase.name)")
}
}
// Registering the observer
TestRunner.shared.addObserver(MyTestObserver())
In this example:
- The
MyTestObserver
class implements theTestObserver
protocol. - It provides hooks for the start and finish of test suites and test cases, logging messages to the console.
Custom Test Execution
SwiftTesting allows for custom test execution strategies, enabling you to define how tests should be run, retried, or skipped based on custom logic.
Example of Custom Test Execution:
@Suite struct CustomExecutionTests {
@Test func testRetryOnFailure() {
var retryCount = 0
while retryCount < 3 {
do {
try performFlakyOperation()
break
} catch {
retryCount += 1
if retryCount == 3 {
Issue.record("Test failed after 3 retries")
}
}
}
#expect(retryCount < 3)
}
}
In this example:
- The test retries the operation up to three times before recording an issue.
- This custom logic helps handle flaky tests that might fail intermittently.
Detailed Test Reporting
Generating detailed test reports is crucial for understanding the outcome of your test runs. SwiftTesting can be integrated with various reporting tools to produce comprehensive test reports.
Example of Generating a Test Report:
@Suite struct ReportingTests {
@Test func testWithReporting() {
let result = performOperation()
if result != .expected {
Issue.record("Operation did not return expected result")
}
#expect(result == .expected)
}
}
// Conceptual example of generating a report
let report = TestReport.generate(for: ReportingTests.self)
print(report.summary)
In this example:
- A test suite named
ReportingTests
is defined with a test that records an issue if the result is not as expected. - A conceptual
TestReport
class generates a summary report for the test suite.
9. Compatibility
SwiftTesting is designed to be highly compatible with various platforms and versions of Swift. This ensures that you can integrate it seamlessly into your existing development environment and leverage its advanced features across different projects.
Supported Platforms
SwiftTesting supports a wide range of platforms, making it versatile for different development needs. The supported platforms include:
- iOS: SwiftTesting can be used to write tests for iOS applications, ensuring that your mobile apps are robust and reliable.
- macOS: For desktop applications, SwiftTesting provides the tools needed to test macOS apps thoroughly.
- visionOS: Support for visionOS allows developers to write tests for applications targeting Apple’s augmented reality platform.
- watchOS: Ensure your watchOS apps are working as expected by leveraging SwiftTesting’s features.
- tvOS: Test your tvOS applications to provide a seamless experience on Apple TV.
- Linux: SwiftTesting also supports Linux, making it a good choice for server-side Swift applications.
Version Compatibility
SwiftTesting is compatible with multiple versions of Swift, ensuring that you can use it regardless of the specific version you are working with. The supported Swift versions include:
- Swift 5.7: SwiftTesting is fully compatible with Swift 5.7, allowing you to leverage the latest language features and improvements.
- Swift 5.8: Continued support for Swift 5.8 ensures that you can upgrade your projects without losing compatibility with SwiftTesting.
- Swift 5.9: SwiftTesting supports the advancements in Swift 5.9, providing access to new language enhancements and optimizations.
- Swift 5.10: Future-proof your projects with compatibility for Swift 5.10, allowing you to take advantage of upcoming features and improvements.
Example of Version-Specific Features
To illustrate how SwiftTesting maintains compatibility across different Swift versions, consider the following example where we use features introduced in Swift 5.7 and later:
import Testing
@Suite struct VersionSpecificTests {
@Test func testNewFeature() {
if #available(swift 5.9) {
// Use features available in Swift 5.9
let result = newSwift59Feature()
#expect(result == expectedOutcome)
} else {
// Fallback for earlier versions
let result = legacyFeature()
#expect(result == expectedOutcome)
}
}
}
In this example:
- The
testNewFeature
function uses a conditional compilation check to determine if a Swift 5.9 feature is available. - This ensures that the test can run on both newer and older versions of Swift, maintaining compatibility and leveraging new language features when available.
10. Comparing XCTest and SwiftTesting
SwiftTesting introduces numerous improvements and changes compared to the traditional XCTest framework. In this section, we’ll compare XCTest and SwiftTesting, highlighting their differences, performance improvements, and the simplified syntax and organization that SwiftTesting offers.
Side-by-Side Code Comparison
To better understand the differences between XCTest and SwiftTesting, let’s look at some side-by-side code comparisons.
Basic Test Case:
XCTest:
import XCTest
class ArithmeticTests: XCTestCase {
func testAddition() {
let result = 1 + 1
XCTAssertEqual(result, 2)
}
}
SwiftTesting:
import Testing
@Suite struct ArithmeticTests {
@Test func testAddition() {
let result = 1 + 1
#expect(result == 2)
}
}
Assertions:
XCTest:
XCTAssertTrue(condition)
XCTAssertFalse(condition)
XCTAssertEqual(value1, value2)
XCTAssertNil(optionalValue)
SwiftTesting:
#expect(condition)
#expect(!condition)
#expect(value1 == value2)
#expect(optionalValue == nil)
Async Testing:
XCTest:
func testAsyncOperation() async throws {
let result = try await asyncFunction()
XCTAssertEqual(result, expectedValue)
}
SwiftTesting:
@Test func testAsyncOperation() async {
let result = await asyncFunction()
#expect(result == expectedValue)
}
Performance Improvements
SwiftTesting provides several performance improvements over XCTest, including:
-
Parallel Execution:
- SwiftTesting runs tests in parallel by default, significantly reducing the time required to execute large test suites. XCTest supports parallel execution, but it requires additional configuration and only runs tests in separate processes.
-
Randomized Test Execution:
- By running tests in random order, SwiftTesting helps identify dependencies between tests that could cause flakiness. XCTest does not have built-in support for randomized test execution.
-
Simplified Setup and Teardown:
- SwiftTesting uses
init
anddeinit
for setup and teardown, providing a more integrated and intuitive approach. XCTest requiressetUp
andtearDown
methods, which can be less flexible
- SwiftTesting uses
.
Simplified Syntax and Organization
SwiftTesting streamlines the syntax and organization of tests, making them easier to write and maintain.
Unified Assertions:
- SwiftTesting consolidates various assertion types into a single
#expect
macro, reducing the need for multipleXCTAssert
functions and making the code cleaner and more readable.
Suite-Based Organization:
- Tests are organized using the
@Suite
macro, allowing for better modularity and separation of test concerns. This contrasts with XCTest’s class-based organization, which can become cumbersome with large test suites.
Traits for Enhanced Metadata:
- SwiftTesting introduces traits like
@Tag
,@Description
,@Bug
, and@Toggle
, providing enhanced metadata and control over test execution. XCTest lacks such built-in metadata handling capabilities.
Example of Traits in SwiftTesting:
@Suite struct FeatureTests {
@Test(.enabled(if: FeatureFlag.isFeatureEnabled))
@Tag("Feature")
@Description("Tests the new feature under condition X")
func testNewFeature() {
let result = performFeatureOperation()
#expect(result == expectedOutcome)
}
}
Migrating from XCTest to SwiftTesting
Migrating your tests from XCTest to SwiftTesting involves several steps, including changing imports, updating test functions, and using new macros for assertions and setup/teardown. Here is a brief overview of the migration process:
- Change Imports:
XCTest:
import XCTest
SwiftTesting:
import Testing
- Update Test Functions:
XCTest:
class MyTests: XCTestCase {
func testExample() {
XCTAssertEqual(2 + 2, 4)
}
}
SwiftTesting:
@Suite struct MyTests {
@Test func testExample() {
#expect(2 + 2 == 4)
}
}
- Use New Macros for Assertions:
XCTest:
XCTAssertNotNil(someValue)
SwiftTesting:
#expect(someValue != nil)
- Update Setup and Teardown:
XCTest:
class MyTests: XCTestCase {
override func setUp() {
super.setUp()
// Setup code here
}
override func tearDown() {
// Teardown code here
super.tearDown()
}
}
SwiftTesting:
@Suite struct MyTests {
init() {
// Setup code here
}
deinit {
// Teardown code here
}
}
11. Conclusion
SwiftTesting represents a significant step forward in the realm of testing frameworks for Swift, offering a modern and efficient alternative to the traditional XCTest framework. With its streamlined syntax, advanced features, and enhanced performance capabilities, SwiftTesting is poised to revolutionize the way developers write and execute tests for Swift applications.
Key Takeaways
- Modern Approach: SwiftTesting introduces a more intuitive and concise way of writing tests, reducing boilerplate and making test code easier to read and maintain.
- Enhanced Performance: With built-in support for parallel and randomized test execution, SwiftTesting significantly improves the speed and reliability of test suites.
- Unified Assertions: The
#expect
macro consolidates various assertion types into a single function, simplifying the syntax and making the test code cleaner. - Flexible Organization: The
@Suite
macro allows for better modularity and organization of test functions, making it easier to manage large test suites. - Advanced Features: Traits, parametrized tests, async testing, and error handling provide powerful tools for writing robust and comprehensive tests.
- Compatibility: SwiftTesting supports a wide range of platforms and Swift versions, ensuring that it can be seamlessly integrated into existing development environments.
Next Steps
As you start exploring and adopting SwiftTesting in your projects, here are a few steps you can take:
- Experiment with Basic Tests: Begin by writing simple test cases using the
@Test
and#expect
macros to get familiar with the new syntax and features. - Organize Tests with Suites: Group related tests into suites using the
@Suite
macro to improve the modularity and readability of your test code. - Leverage Advanced Features: Explore the use of traits, parametrized tests, async testing, and detailed error handling to enhance the robustness and coverage of your test suite.
- Integrate with CI/CD: Ensure that your tests run as part of your continuous integration and delivery pipelines to catch issues early and maintain high code quality.
References and Further Reading
- SwiftTesting GitHub Repository
- Swift Package Index Documentation
- Official Swift Testing Overview
- WWDC24 Announcement
By embracing SwiftTesting, you are taking a significant step towards more efficient, reliable, and maintainable testing practices in your Swift development projects. We look forward to seeing how you leverage this powerful new tool to enhance your software quality and development workflow.